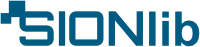 |
SIONlib
1.7.6
Scalable I/O library for parallel access to task-local files
|
Go to the documentation of this file.
14 #ifndef SION_SION_FILE_H
15 #define SION_SION_FILE_H
22 #define SION_FILE_FLAG_ANSI 1
23 #define SION_FILE_FLAG_SCNDANSI 2
24 #define SION_FILE_FLAG_POSIX 4
25 #define SION_FILE_FLAG_CREATE 8
26 #define SION_FILE_FLAG_WRITE 16
27 #define SION_FILE_FLAG_READ 32
28 #define SION_FILE_FLAG_SIONFWD 64
30 struct _sion_fileptr_s {
93 #if defined(_SION_SIONFWD)
94 int _sion_file_open_sionfwd_write_create(
const char *fname,
unsigned int addflags);
95 int _sion_file_open_sionfwd_write_existing(
const char *fname,
unsigned int addflags);
96 int _sion_file_open_sionfwd_read(
const char *fname,
unsigned int addflags);
97 int _sion_file_close_sionfwd(
int fd);
98 long _sion_file_get_opt_blksize_sionfwd(
int fd);
99 int _sion_file_flush_sionfwd(
int fd);
100 int _sion_file_purge_sionfwd(
int fd);
101 int _sion_file_set_buffer_sionfwd(
int fd,
char *buffer, sion_int32 buffer_size);
102 sion_int64 _sion_file_write_sionfwd(
const void *data, sion_int64 bytes,
int fd, sion_int64 *position);
103 sion_int64 _sion_file_read_sionfwd(
void *data, sion_int64 bytes,
int fd, sion_int64 *position);
sion_int64 _sion_file_get_position_posix(int fd)
POSIX: Get the current position in file.
char * _sion_get_fileptr_desc(_sion_fileptr *sion_fileptr)
int _sion_file_flush(_sion_fileptr *fileptr)
Flush data to file.
long _sion_file_get_opt_blksize_posix(int fd)
POSIX: Get optional file system block size for a file.
int _sion_file_stat_file(const char *fname)
Check if file exists (LARGE_FILE support on BlueGene)
int _sion_file_get_fd(_sion_fileptr *sion_fileptr)
Utility function: Get POSIX fp.
int _sion_file_set_second_fileptr(_sion_fileptr *sion_fileptr, FILE *fileptr)
Set second fileptr for file if opened with ANSI.
sion_int64 _sion_file_write_posix(const void *data, sion_int64 bytes, int fd)
POSIX: Write data to file.
sion_int64 _sion_file_read(void *data, sion_int64 bytes, _sion_fileptr *sion_fileptr)
Read data from file.
_sion_fileptr * _sion_file_open(const char *fname, unsigned int flags, unsigned int addflags)
Create and open a new file for writing.
int _sion_file_set_buffer_ansi(FILE *fileptr, char *buffer, sion_int32 buffer_size)
ANSI: set buffer of fp.
sion_int64 _sion_file_write(const void *data, sion_int64 bytes, _sion_fileptr *sion_fileptr)
Write data to file.
int _sion_file_purge_ansi(FILE *fileptr)
ANSI: Purge the data to the disk.
int _sion_file_open_posix_read(const char *fname, unsigned int addflags)
POSIX: Open a file for reading.
int _sion_file_close_ansi(FILE *fileptr)
ANSI: Close a file.
sion_int64 _sion_file_read_ansi(void *data, sion_int64 bytes, FILE *fileptr)
ANSI: Read data from file.
int _sion_file_close_posix(int fd)
POSIX: Close a file.
int _sion_file_flush_posix(int fd)
POSIX: Flush the data to the disk.
sion_int64 _sion_file_set_position_posix(int fd, sion_int64 startpointer)
POSIX: Set the start position for the current task.
long _sion_file_get_opt_blksize(_sion_fileptr *fileptr)
Get optional file system block size for a file.
int _sion_file_set_buffer(_sion_fileptr *fileptr, char *buffer, sion_int32 buffer_size)
Set buffer of fp.
FILE * _sion_file_open_ansi_write_create(const char *fname, unsigned int addflags)
ANSI: Create and open a new file for writing.
FILE * _sion_file_open_ansi_write_existing(const char *fname, unsigned int addflags)
ANSI: Open a new file for writing.
int _sion_file_set_buffer_posix(int fd, char *buffer, sion_int32 buffer_size)
POSIX: set buffer of fd.
int _sion_file_unset_second_fileptr(_sion_fileptr *sion_fileptr)
Unset second fileptr for file if opened with ANSI.
sion_int64 _sion_file_read_posix(void *data, sion_int64 bytes, int fd)
POSIX: Read data from file.
int _sion_file_open_posix_write_existing(const char *fname, unsigned int addflags)
POSIX: Open a new file for writing.
int _sion_file_flush_ansi(FILE *fileptr)
ANSI: Flush the data to the disk.
sion_int64 _sion_file_write_ansi(const void *data, sion_int64 bytes, FILE *fileptr)
ANSI: Write data to file.
int _sion_file_open_posix_write_create(const char *fname, unsigned int addflags)
POSIX: Create and open a new file for writing.
FILE * _sion_file_open_ansi_read(const char *fname, unsigned int addflags)
ANSI: Open a file for reading.
int _sion_file_stat_file2(const char *fname, unsigned int apiflag)
Check if file exists with appropriate low-level API.
int _sion_file_close(_sion_fileptr *sion_fileptr)
Close file and destroys fileptr structure.
sion_int64 _sion_file_get_position_ansi(FILE *fileptr)
ANSI: Get the current position in file.
_sion_fileptr * _sion_file_alloc_and_init_sion_fileptr(void)
Create and return _sion_fileptr.
int _sion_file_purge(_sion_fileptr *fileptr)
Purge data to file.
sion_int64 _sion_file_set_position(_sion_fileptr *fileptr, sion_int64 startpointer)
Set new position in file.
sion_int64 _sion_file_set_position_ansi(FILE *fileptr, sion_int64 startpointer)
ANSI: Set the start position for the current task.
int _sion_file_purge_posix(int fd)
POSIX: Purge the data to the disk.
long _sion_file_get_opt_blksize_ansi(FILE *fileptr)
ANSI: Get optional file system block size for a file.
sion_int64 _sion_file_get_position(_sion_fileptr *fileptr)
Get new position in file.